Getting User Input With a VBA Input Box
In this lesson we'll see how to get input from a user by using a VBA input box. You will learn how to write a sentence in the document a certain amount of times This could be useful if you wanted to fill up a page with text and print it to see if the margins etc. look OK. 1. Open a new document in Word. 2. Open the VBA Editor (Tools>Macro>Visual Basic Editor). 3. Copy the following text from Sub InputBoxInsertText() to End Sub, inclusively. Sub InputBoxInsertText() Selection.HomeKey wdStory TheSentence = InputBox("Type Text Please", "Using the VBA Input Box") HowManyTimes = InputBox("How many times", "Using the VBA Input Box") For Counter = 1 To HowManyTimes Selection.TypeText TheSentence & " " Next End Sub 4. Put the cursor anywhere within this code block. 5. Press F5 6. Type a word or two into the first VBA input box that appears. 7. Type a number (between 50 and 100) into the second Input Box. 8. Go to the new document you opened and see how the word or two that you typed into the input box appears repeated the amount of times corresponding to value you entered into the second input box. Let’s examine these 6 lines of code... Selection.HomeKey wdStory I recorded this with the Macro Recorder. Its purpose is to make sure the cursor is at the beginning of the document before our words are typed. It says, where the Selection is, press the Home key and wdStory (Word story) says that we are holding down the Control (CTRL) key while pressing the Home key, which, CTRL + the Home Key, will move the cursor from wherever it is to the beginning of the document. In the next line... TheSentence = InputBox("Type Text Please", "Using the VBA Input Box") we will learn two new concepts. The first is about variables, the word TheSentence is not a Keyword in VBA, as the word Sub and End are, nor is it a Keyword in Word, as Selection and HomeKey are. It is a word that we created and is considered a "variable" by VBA. A variable can hold anything, a string, number, date, and other things. For simplicity, we’ll leave the details of a variable until a little later. The second new concept is a Function. For our purpose in this example we’ll look at only two properties of a function. In a previous tutorial we used a message box (MsgBox) to send us a popup message saying "Done" after all the previous lines of code were executed. Msgbox is also a function, as is InputBox is this example. There is a difference between how we used the MsgBox function earlier and the way we are using InputBox in this example. It is slight, can you spot it? MsgBox "Done"
TheSentence = InputBox("Type Text Please", "Using the VBA Input Box") The difference is the equal sign before the function. Using VBA input box without an equal sign before it is useless because we would lose whatever is typed into it by the user. In our case, whatever is typed into the input box will be stored in the variable "TheSentence." On the other hand, MsgBox can be used both ways. MsgBox "Done" (without an equal sign was used to tell us the code was virtually finished running. The only statement left was the End Sub line which doesn’t perform any task. If we had been asking the user if he wanted to save the document, for example, we would have wanted to know if he had clicked Yes or No. And either saved the document for him, or not. -----SIDEBAR----- Although the message box we saw had only an OK button, the middle argument that we skipped over controls which buttons are displayed by the message box. -----SIDEBAR----- Her answer would have been entered into the variable to the left of an equal sign. Along with this point, the is a syntax issue with using a function to the right of an equal sign. If we were asking if the user wanted to save the document, typing: TheAnswer = MsgBox "Do you want to save the document?" would have yielded a syntax error. When using a function to the left of an equal sign, we must enclose the function’s arguments in parentheses: TheAnswer = MsgBox("Do you want to save the document?") For the VBA input box, only the first argument is mandatory. The second argument is to give the InputBox’s window a Title, so I added this after a comma. The first argument is the message the user will see as she is prompted for input. TheSentence = InputBox("Type Text Please", "Using the VBA Input Box") 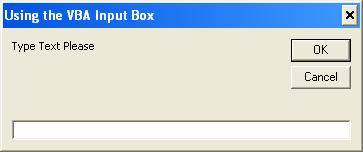 The next line... HowManyTimes = InputBox("How many times", "Using the VBA Input Box") is a repetition of the previous line with the purpose of acquiring the value of the number of times to repeat the typing of the text. The next three lines comprise what is called a loop... For Counter = 1 To HowManyTimes Selection.TypeText TheSentence & " " Next Just as Sub () and End Sub enclose a block of code, For and Next are one way to create a loop. Counter is not a Keyword and thereby considered a variable. "To" is a Keyword. This line is saying to keep executing the code between itself and the Keyword Next while counting from 1 "To" HowManyTimes, which is the variable that is now holding the second input box’s answer as to how many times to type the text that was entered into the first input box. Congratulations are in order if you have gone though these four beginning tutorials. Again, please let us know if anything in our tutorials was not clear, went over your head or showed up more than minimally different in the version of your Visual Basic Editor by letting us know the version of Word or Office that you are using. Thank you. By the way, if you should want to create this particular macro at a later time on a different computer because you either want to see how a page margins look printed or how many kilobytes a 100 page document would be, there is a built-in way to do this in Word. Type... "=rand(number of paragraphs,number of sentences per paragraph)" (without the quotes) and press Enter. Word will type the sentence, "The quick brown fox jumps over the lazy dog." In that many paragraphs, that many times. Typing: =rand(2,5) and then pressing Enter will yield: 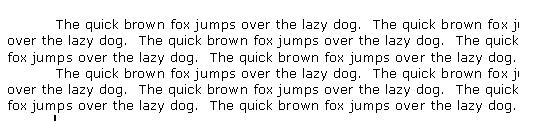 Return from VBA Input box to "Free VBA Tutorials" Return to our Homepage  |